6: Histograms
In nearly every High Energy Physics analysis, histograms have their place: - plotting of variables - calculating efficiency correction tables (weights) - performing binned fits
and many more.
Scikit-HEP offers libraries to deal with histograms in Python in a performant way: hist is a user-friendly analysis library for histograms and is directly built on top of the workhorse boost-histogram, which can also be used directly. Hist simply provides more functionality.
They are written by the same authors and can also be used often together.
Both also work well together with mplhep, the plotting library.
[1]:
# jupyter magic to load the previous data sets
%store -r bkg_df
%store -r mc_df
%store -r data_df
import boost_histogram as bh
import hist
import mplhep
import numpy as np
[2]:
# Let's get started with a simple example
# Compose axis however you like; this is a 2D histogram
h = bh.Histogram(bh.axis.Regular(2, 0, 1),
bh.axis.Regular(4, 0.0, 1.0))
# Filling can be done with arrays, one per dimension
h.fill([.3, .5, .2],
[.1, .4, .9])
# NumPy array view into histogram counts, no overflow bins
counts = h.view()
variances = h.variances()
mplhep.hist2dplot(h)
[2]:
ColormeshArtists(pcolormesh=<matplotlib.collections.QuadMesh object at 0x7efc85743490>, cbar=<matplotlib.colorbar.Colorbar object at 0x7efc8575e1d0>, text=[])
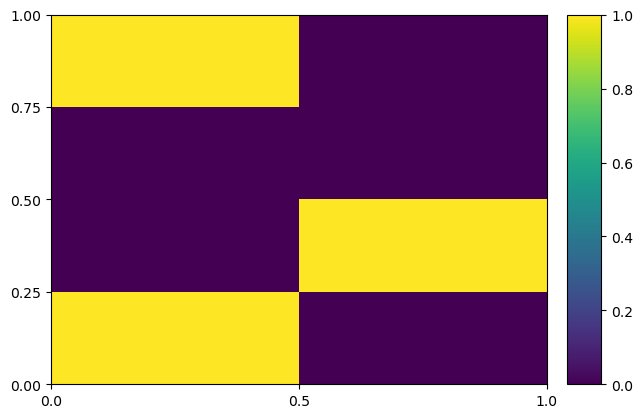
Axes
A cental part of a histogram are the axes: They difine the binning and other treats of the axis.
A Hist (this refers by default to hist.Hist, but usually also applies for bh.Histogram as the former inherits from the latter) can have multiple axes of different types.
All axes are described here.
The most important types are
Regular
This is an axis with lower, upper limits, regularly split into n bins.
axis_reg = hist.axis.Regular(nbins, lower, upper, name=name)
Variable
A variable axis allows to set the bin edges arbitrarily using an array-like object.mro
axis_var = hist.axis.Variable([0, 0.5, 3.1, 3.4], name="eta")
Axis Name
An axis (in hist, in bh only a label is possible) have a name, which can be used as the identifier when working with the histogram (instead of using plain integer indexes).
[ ]:
[3]:
start, stop = data_df['Jpsi_M'].min(), data_df['Jpsi_M'].max()
axis1 = hist.axis.Regular(bins=50, start=start, stop=stop, name="mass")
To create a histogram, we can pass one or multiple axes to a histogram
[4]:
data_h = hist.Hist(axis1)
[5]:
data_h.fill(data_df['Jpsi_M'])
[5]:
Double() Σ=168384.0 (168385.0 with flow)
[6]:
mc_h = hist.Hist(axis1).fill(mc_df['Jpsi_M']) # we can also chain the commands
Compatibility with mplhep
With bh and hist, the Unified Histogram Interface was also born. This allows objects to be plotted so that a library such as mplhep knows what to do with it.
In short, mplhep and hist work seemless together:
[7]:
mplhep.histplot(data_h)
[7]:
[StairsArtists(stairs=<matplotlib.patches.StepPatch object at 0x7efc855ea450>, errorbar=<ErrorbarContainer object of 3 artists>, legend_artist=<ErrorbarContainer object of 3 artists>)]
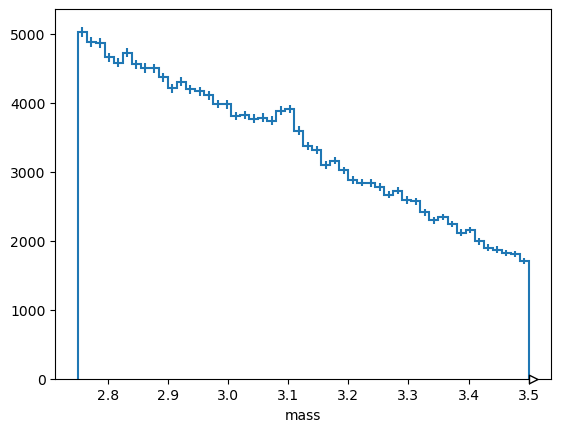
Plotting with hist
hist itself provides also plotting functionality
[8]:
data_h.plot1d()
[8]:
[StairsArtists(stairs=<matplotlib.patches.StepPatch object at 0x7efc57d6d010>, errorbar=<ErrorbarContainer object of 3 artists>, legend_artist=<ErrorbarContainer object of 3 artists>)]
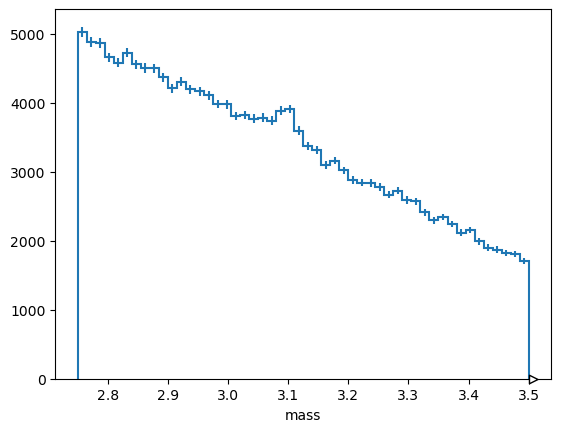
[9]:
data_h.plot1d()
mc_h.plot1d()
[9]:
[StairsArtists(stairs=<matplotlib.patches.StepPatch object at 0x7efc57bbb990>, errorbar=<ErrorbarContainer object of 3 artists>, legend_artist=<ErrorbarContainer object of 3 artists>)]
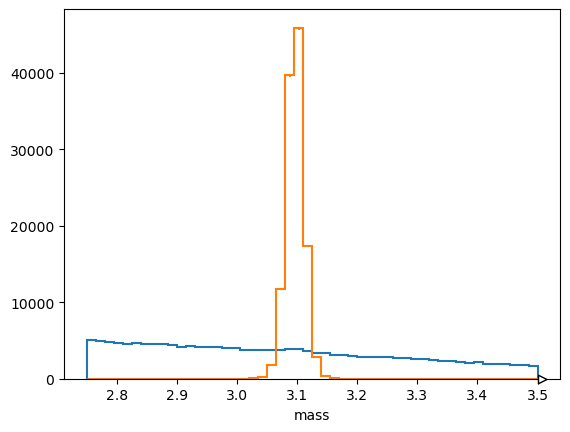
[10]:
mc_df.columns
[10]:
Index(['Jpsi_PE', 'Jpsi_PX', 'Jpsi_PY', 'Jpsi_PZ', 'Jpsi_PT', 'Jpsi_P',
'Jpsi_M', 'mum_PT', 'mum_PX', 'mum_PY', 'mum_PZ', 'mum_IP', 'mum_eta',
'mum_M', 'mum_PE', 'mup_PT', 'mup_PX', 'mup_PY', 'mup_PZ', 'mup_IP',
'mup_eta', 'mup_M', 'mup_PE', 'nTracks', 'mum_ProbNNmu', 'mum_ProbNNpi',
'mup_ProbNNmu', 'mup_ProbNNpi', 'Jpsi_eta', 'mup_P', 'mum_P',
'catagory', 'BDT'],
dtype='object')
Multiple dimensions
Histograms can be multiple dimensional. Let’s add a dimension to it.
[11]:
start, stop = data_df['BDT'].min(), data_df['BDT'].max()
axis_bdt = hist.axis.Regular(bins=20, start=start, stop=stop, name="BDT")
[12]:
mc_h2d = hist.Hist(axis1, axis_bdt).fill(BDT=mc_df['BDT'], mass=mc_df['Jpsi_M']) # using names
[13]:
data_h2d = hist.Hist(axis1, axis_bdt)
data_h2d.fill(data_df['Jpsi_M'], data_df['BDT']) # order based
[13]:
Regular(20, 0.026503, 0.993653, name='BDT')
Double() Σ=168383.0 (168385.0 with flow)
[14]:
mplhep.hist2dplot(data_h2d)
[14]:
ColormeshArtists(pcolormesh=<matplotlib.collections.QuadMesh object at 0x7efc57a64790>, cbar=<matplotlib.colorbar.Colorbar object at 0x7efc57c1c210>, text=[])
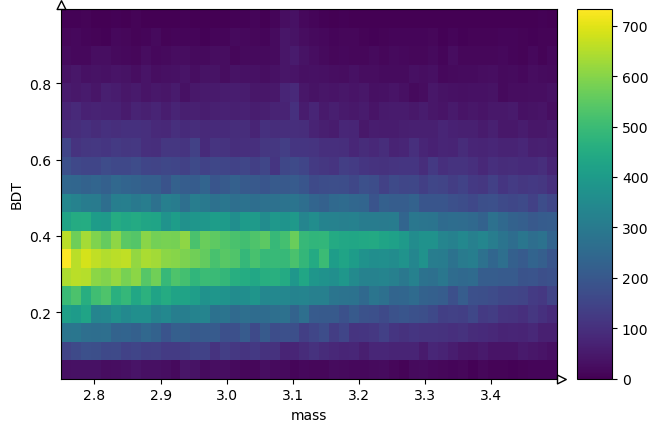
Access Bins
hist allows you to access the bins of your Hist by various ways. Besides the normal access by index, you can use locations (supported by boost-histogram), complex numbers, and the dictionary to access the bins.
[15]:
# Access by bin number
data_h2d[35, 5]
[15]:
318.0
Getting Density
If you want to get the density of an existing histogram, .density() is capable to do it and will return you the density array without overflow and underflow bins.
A histogram is a count, so it’s an integral over a density. To obtain the density, one can devide by the area of the bin, this gives the “average density” in a bin.
[16]:
data_h2d.density()
[16]:
array([[0.24562342, 1.20355474, 2.32523501, 3.37322826, 4.07734872,
5.27271602, 6.00139882, 5.38734028, 3.48785252, 2.77554461,
1.97317478, 1.4737405 , 1.21992964, 0.7286828 , 0.6058711 ,
0.42574726, 0.2947481 , 0.17193639, 0.09824937, 0.02456234],
[0.27018576, 1.34274135, 2.36617225, 3.26679145, 4.2984098 ,
5.37915283, 5.37915283, 4.68321982, 3.66797636, 2.66092035,
1.94861244, 1.30999156, 0.87605685, 0.76143259, 0.69593302,
0.39299747, 0.35206023, 0.13099916, 0.13099916, 0.02456234],
[0.26199831, 1.46555306, 2.16148607, 3.49603997, 3.76622573,
5.33002815, 5.6165888 , 5.19084155, 3.68435126, 2.48898396,
1.85855052, 1.21992964, 0.95793133, 0.83511962, 0.54855896,
0.42574726, 0.27837321, 0.12281171, 0.08187447, 0.05731213],
[0.27018576, 1.43280327, 2.14511118, 2.74279482, 4.16741064,
4.95340558, 5.42827752, 4.83059387, 3.29135379, 2.47260907,
1.9240501 , 1.23630453, 0.98249367, 0.7286828 , 0.56493386,
0.33568534, 0.2947481 , 0.22106108, 0.06549958, 0.01637489],
[0.20468618, 1.32636645, 2.16148607, 2.76735717, 4.32297214,
4.86334366, 5.31365326, 4.576783 , 3.24222911, 2.13692373,
1.82580073, 1.36730369, 0.92518154, 0.82693217, 0.55674641,
0.50762173, 0.27837321, 0.22924852, 0.04093724, 0.01637489],
[0.22924852, 1.35911624, 1.89130031, 3.02116803, 3.79897552,
5.05984239, 5.37915283, 4.98615537, 3.66797636, 2.53810864,
2.00592457, 1.30180411, 1.0234309 , 0.78599493, 0.54855896,
0.4503096 , 0.32749789, 0.1555615 , 0.13099916, 0.03274979],
[0.24562342, 1.1953673 , 1.89948776, 2.8246693 , 3.9872868 ,
4.78146919, 5.42827752, 4.46215874, 3.56972699, 2.57085843,
1.91586265, 1.29361666, 0.94155643, 0.84330707, 0.4339347 ,
0.36843513, 0.28656065, 0.1391866 , 0.10643681, 0.00818745],
[0.31112299, 1.22811709, 1.82580073, 2.95566845, 3.57791444,
4.96978047, 5.03528005, 4.38847172, 3.65160147, 2.43167183,
1.85855052, 1.36730369, 0.98249367, 0.82693217, 0.58130875,
0.40118491, 0.22924852, 0.11462426, 0.04912468, 0.04912468],
[0.24562342, 1.12986772, 1.99773713, 2.9638559 , 3.82353786,
4.42122151, 5.23177878, 4.92884324, 3.52060231, 2.5135463 ,
1.76030116, 1.21992964, 0.81874472, 0.81055728, 0.51580918,
0.31931044, 0.31931044, 0.19649873, 0.09006192, 0. ],
[0.25381086, 1.15443006, 1.87492542, 2.68548269, 3.48785252,
4.70778216, 5.17446665, 4.7323445 , 3.50422742, 2.2187982 ,
1.7684886 , 1.20355474, 0.77780749, 0.69593302, 0.6058711 ,
0.39299747, 0.22106108, 0.1391866 , 0.1555615 , 0.00818745],
[0.22106108, 1.03161835, 1.54742753, 2.79191951, 3.66797636,
4.02003659, 4.96159302, 4.78146919, 3.08666761, 2.56267098,
1.53924008, 1.27724177, 0.95793133, 0.67955812, 0.49124683,
0.34387278, 0.25381086, 0.14737405, 0.04912468, 0.01637489],
[0.1555615 , 1.0398058 , 1.79305094, 2.55448354, 3.48785252,
4.31478469, 4.92065579, 4.74053195, 3.30772868, 2.49717141,
1.84217563, 1.21992964, 0.94974388, 0.81055728, 0.59768365,
0.4339347 , 0.27018576, 0.19649873, 0.08187447, 0.00818745],
[0.33568534, 1.05618069, 1.80123839, 2.54629609, 3.47147763,
4.21653533, 4.81421897, 4.97796792, 3.01298058, 2.16967352,
1.75211371, 1.17080495, 0.90880664, 0.72049536, 0.49943428,
0.27837321, 0.31931044, 0.19649873, 0.08187447, 0.03274979],
[0.28656065, 1.18717985, 1.66205179, 2.67729525, 3.33229102,
3.92178723, 4.69959471, 4.27384746, 3.16035463, 2.5299212 ,
1.72755137, 1.34274135, 1.17080495, 0.76962004, 0.53218407,
0.39299747, 0.21287363, 0.1555615 , 0.08187447, 0. ],
[0.17193639, 1.18717985, 1.71117647, 2.70185759, 3.06210527,
4.08553617, 4.56859556, 4.62590769, 3.16854208, 2.43167183,
1.91586265, 1.18717985, 0.71230791, 0.69593302, 0.48305939,
0.41755981, 0.30293555, 0.14737405, 0.03274979, 0.01637489],
[0.22924852, 0.8842443 , 1.71117647, 2.24336054, 3.15216719,
4.06097383, 4.26566001, 4.51128343, 3.31591613, 2.2187982 ,
1.57198987, 1.26905432, 0.9006192 , 0.72049536, 0.51580918,
0.40118491, 0.30293555, 0.19649873, 0.09006192, 0.01637489],
[0.21287363, 1.09711793, 1.45736561, 2.30886012, 3.07848016,
3.96272446, 4.4375964 , 4.37209682, 3.26679145, 2.34979736,
1.67842668, 1.22811709, 0.87605685, 0.71230791, 0.55674641,
0.46668449, 0.20468618, 0.19649873, 0.08187447, 0.01637489],
[0.16374894, 0.96611877, 1.46555306, 2.04686181, 2.89835632,
3.75803828, 4.35572193, 4.27384746, 2.93110611, 2.17786096,
1.81761329, 1.1789924 , 0.77780749, 0.75324515, 0.52399662,
0.49124683, 0.28656065, 0.10643681, 0.14737405, 0.03274979],
[0.10643681, 0.9006192 , 1.69480158, 2.16148607, 2.93110611,
3.6925387 , 3.99547425, 4.18378554, 3.27497889, 2.25154799,
1.68661413, 1.25267943, 0.80236983, 0.76962004, 0.54037152,
0.4503096 , 0.26199831, 0.16374894, 0.12281171, 0.02456234],
[0.09824937, 0.99068112, 1.35092879, 2.08779905, 3.12760484,
3.58610189, 4.25747256, 4.31478469, 3.25041655, 2.15329862,
1.65386434, 1.10530538, 0.81874472, 0.6058711 , 0.49124683,
0.36843513, 0.27837321, 0.14737405, 0.09824937, 0.08187447],
[0.22106108, 0.85149451, 1.67023924, 2.09598649, 2.76735717,
3.76622573, 4.02822404, 4.48672109, 2.90654377, 2.22698565,
1.58017732, 1.25267943, 0.94155643, 0.82693217, 0.50762173,
0.36024768, 0.22924852, 0.1555615 , 0.09006192, 0.00818745],
[0.16374894, 0.85149451, 1.38367858, 2.17786096, 2.80010695,
3.78260062, 4.05278638, 4.01184915, 3.19310442, 2.2187982 ,
1.68661413, 0.91699409, 0.94974388, 0.75324515, 0.58130875,
0.38481002, 0.26199831, 0.18012384, 0.17193639, 0.09006192],
[0.1555615 , 0.6058711 , 1.4737405 , 2.21061075, 2.78373206,
3.64341402, 4.02822404, 4.21653533, 3.1030425 , 2.29248523,
1.65386434, 1.27724177, 1.08893048, 0.79418238, 0.57312131,
0.61405854, 0.34387278, 0.34387278, 0.34387278, 0.22924852],
[0.09006192, 0.63043344, 1.43280327, 1.89130031, 2.69367014,
3.17672953, 4.2820349 , 4.63409514, 3.23404166, 2.31704757,
1.69480158, 1.35911624, 0.93336898, 0.78599493, 0.82693217,
0.68774557, 0.32749789, 0.42574726, 0.30293555, 0.27837321],
[0.13099916, 0.73687025, 1.11349282, 2.16148607, 2.71004503,
3.5533521 , 3.97091191, 4.01184915, 2.74279482, 2.14511118,
1.57198987, 1.21992964, 0.93336898, 0.76143259, 0.46668449,
0.35206023, 0.23743597, 0.28656065, 0.18831129, 0.13099916],
[0.10643681, 0.85968196, 1.25267943, 1.69480158, 2.57904588,
3.14397974, 3.65160147, 3.93816212, 2.91473122, 1.94042499,
1.35911624, 1.0234309 , 0.90880664, 0.58130875, 0.62224599,
0.30293555, 0.26199831, 0.22106108, 0.14737405, 0.09006192],
[0.10643681, 0.73687025, 1.38367858, 1.67023924, 2.59542077,
3.04573037, 4.10191107, 3.94634957, 2.67729525, 1.83398818,
1.39186603, 0.94974388, 0.81055728, 0.50762173, 0.40118491,
0.34387278, 0.25381086, 0.1555615 , 0.1391866 , 0.03274979],
[0.17193639, 0.67137067, 1.07255559, 1.68661413, 2.34160991,
2.95566845, 3.30772868, 3.64341402, 2.62817056, 2.03048691,
1.42461582, 0.89243175, 0.81055728, 0.4503096 , 0.49943428,
0.32749789, 0.21287363, 0.10643681, 0.09006192, 0.00818745],
[0.1555615 , 0.62224599, 1.23630453, 1.49830284, 2.29248523,
3.16854208, 3.49603997, 3.56972699, 2.57904588, 2.0796116 ,
1.38367858, 1.08893048, 0.79418238, 0.65499578, 0.40937236,
0.35206023, 0.20468618, 0.1391866 , 0.10643681, 0.04093724],
[0.1391866 , 0.65499578, 0.9006192 , 1.67023924, 2.25154799,
2.84104419, 3.29954124, 3.54516465, 2.61179567, 1.98136223,
1.28542922, 1.04799325, 0.65499578, 0.63043344, 0.37662257,
0.31112299, 0.27018576, 0.11462426, 0.10643681, 0.01637489],
[0.13099916, 0.50762173, 0.93336898, 1.52286519, 2.03048691,
2.66092035, 3.06210527, 3.60247678, 2.49717141, 1.93223755,
1.4737405 , 0.93336898, 0.7450577 , 0.39299747, 0.44212215,
0.35206023, 0.20468618, 0.10643681, 0.05731213, 0. ],
[0.1555615 , 0.64680833, 0.97430622, 1.4737405 , 2.32523501,
2.6527329 , 2.93110611, 3.62703913, 2.50535885, 1.56380242,
1.38367858, 0.8842443 , 0.67955812, 0.49124683, 0.31112299,
0.24562342, 0.20468618, 0.14737405, 0.04093724, 0.01637489],
[0.17193639, 0.65499578, 1.13805517, 1.35092879, 2.18604841,
2.71004503, 2.95566845, 3.50422742, 2.46442162, 1.81761329,
1.16261751, 0.85968196, 0.75324515, 0.46668449, 0.40937236,
0.27018576, 0.17193639, 0.11462426, 0.06549958, 0. ],
[0.11462426, 0.59768365, 0.93336898, 1.52286519, 1.94042499,
2.59542077, 2.89016887, 3.42235294, 2.49717141, 1.75211371,
1.34274135, 0.90880664, 0.58130875, 0.45849705, 0.44212215,
0.32749789, 0.18012384, 0.1555615 , 0.07368703, 0.00818745],
[0.06549958, 0.59768365, 0.85968196, 1.58836476, 1.94861244,
2.40710949, 3.07848016, 3.25041655, 1.9240501 , 1.75211371,
1.24449198, 0.92518154, 0.61405854, 0.49943428, 0.44212215,
0.23743597, 0.18012384, 0.1391866 , 0.07368703, 0.00818745],
[0.16374894, 0.6058711 , 0.93336898, 1.49830284, 2.0796116 ,
2.60360822, 2.75098227, 2.89835632, 2.3743597 , 1.85855052,
1.28542922, 0.93336898, 0.80236983, 0.52399662, 0.39299747,
0.1555615 , 0.25381086, 0.10643681, 0.08187447, 0. ],
[0.18012384, 0.42574726, 0.87605685, 1.44917816, 1.85855052,
2.26792288, 3.04573037, 3.02935548, 2.32523501, 1.58836476,
1.10530538, 0.76962004, 0.63043344, 0.53218407, 0.46668449,
0.20468618, 0.22924852, 0.10643681, 0.09006192, 0.01637489],
[0.13099916, 0.64680833, 0.8842443 , 1.32636645, 1.81761329,
2.20242331, 2.5299212 , 3.05391782, 2.30886012, 1.58017732,
1.26086687, 1.00705601, 0.54037152, 0.51580918, 0.35206023,
0.35206023, 0.23743597, 0.16374894, 0.11462426, 0.01637489],
[0.10643681, 0.56493386, 0.85968196, 1.12168027, 1.73573881,
2.3743597 , 2.48898396, 2.73460738, 2.0632367 , 1.58836476,
1.20355474, 0.80236983, 0.63043344, 0.61405854, 0.32749789,
0.28656065, 0.12281171, 0.08187447, 0.04093724, 0. ],
[0.07368703, 0.49124683, 0.81055728, 1.15443006, 1.45736561,
2.08779905, 2.26792288, 2.61179567, 2.11236139, 1.4737405 ,
1.06436814, 0.87605685, 0.6058711 , 0.54855896, 0.41755981,
0.27837321, 0.16374894, 0.20468618, 0.07368703, 0.00818745],
[0.11462426, 0.36024768, 0.85968196, 1.11349282, 1.74392626,
2.02229947, 2.47260907, 2.79191951, 2.10417394, 1.38367858,
1.1789924 , 0.8842443 , 0.73687025, 0.51580918, 0.31112299,
0.25381086, 0.17193639, 0.09824937, 0.04093724, 0.01637489],
[0.04093724, 0.33568534, 0.7450577 , 1.26905432, 1.4737405 ,
2.0632367 , 2.54629609, 2.57904588, 2.03867436, 1.30180411,
0.99068112, 0.81055728, 0.62224599, 0.53218407, 0.35206023,
0.27018576, 0.17193639, 0.11462426, 0.06549958, 0.01637489],
[0.10643681, 0.41755981, 0.70412046, 1.01524346, 1.44099071,
1.75211371, 2.2187982 , 2.75916972, 1.87492542, 1.43280327,
0.94155643, 0.67137067, 0.59768365, 0.44212215, 0.31112299,
0.26199831, 0.19649873, 0.11462426, 0.05731213, 0.01637489],
[0.06549958, 0.31112299, 0.66318323, 1.07255559, 1.37549114,
1.75211371, 1.96498734, 2.5299212 , 2.22698565, 1.4737405 ,
1.14624261, 0.80236983, 0.65499578, 0.53218407, 0.38481002,
0.28656065, 0.21287363, 0.11462426, 0.06549958, 0.00818745],
[0.06549958, 0.32749789, 0.59768365, 0.89243175, 1.27724177,
1.69480158, 2.16148607, 2.31704757, 2.02229947, 1.35092879,
0.93336898, 0.7450577 , 0.57312131, 0.38481002, 0.39299747,
0.16374894, 0.1555615 , 0.12281171, 0.09006192, 0.01637489],
[0.04093724, 0.31112299, 0.62224599, 0.87605685, 1.25267943,
1.69480158, 1.81761329, 2.12873628, 1.86673797, 1.28542922,
1.0398058 , 0.76143259, 0.57312131, 0.37662257, 0.40118491,
0.18831129, 0.17193639, 0.07368703, 0.07368703, 0.00818745],
[0.06549958, 0.40937236, 0.59768365, 0.80236983, 1.20355474,
1.53105263, 1.71117647, 2.34979736, 1.79305094, 1.35911624,
0.95793133, 0.72049536, 0.63862088, 0.46668449, 0.26199831,
0.20468618, 0.13099916, 0.06549958, 0.05731213, 0. ],
[0.09006192, 0.36024768, 0.67137067, 0.95793133, 1.0398058 ,
1.55561497, 1.91586265, 2.13692373, 1.65386434, 1.15443006,
0.99068112, 0.70412046, 0.50762173, 0.36024768, 0.2947481 ,
0.21287363, 0.18831129, 0.13099916, 0.04093724, 0. ],
[0.08187447, 0.31112299, 0.52399662, 0.83511962, 0.97430622,
1.43280327, 1.79305094, 2.25973544, 1.71936392, 1.40005348,
0.95793133, 0.77780749, 0.51580918, 0.35206023, 0.31112299,
0.22924852, 0.18012384, 0.06549958, 0.05731213, 0.02456234],
[0.08187447, 0.24562342, 0.53218407, 0.71230791, 1.21174219,
1.48192795, 1.57198987, 1.91586265, 1.75211371, 1.25267943,
0.80236983, 0.6058711 , 0.49124683, 0.40118491, 0.22924852,
0.26199831, 0.1555615 , 0.13099916, 0.06549958, 0.01637489]])
Projecting axes
We can also project onto a certain axis
[17]:
data_h2d.project("mass") # we will here retain the 1D histogram
[17]:
Double() Σ=168384.0 (168385.0 with flow)
Accessing everything relevant
Hist is transparent and let’s us use many things
[18]:
data_h2d.axes
[18]:
(Regular(50, 2.75, 3.5, name='mass'),
Regular(20, 0.026503, 0.993653, name='BDT'))
[19]:
data_h2d.axes['mass']
[19]:
Regular(50, 2.75, 3.5, name='mass')
[20]:
data_h2d.axes['mass'].edges
[20]:
array([2.7500011 , 2.76500103, 2.78000096, 2.79500089, 2.81000082,
2.82500076, 2.84000069, 2.85500062, 2.87000055, 2.88500048,
2.90000041, 2.91500035, 2.93000028, 2.94500021, 2.96000014,
2.97500007, 2.99000001, 3.00499994, 3.01999987, 3.0349998 ,
3.04999973, 3.06499966, 3.0799996 , 3.09499953, 3.10999946,
3.12499939, 3.13999932, 3.15499925, 3.16999919, 3.18499912,
3.19999905, 3.21499898, 3.22999891, 3.24499884, 3.25999878,
3.27499871, 3.28999864, 3.30499857, 3.3199985 , 3.33499843,
3.34999837, 3.3649983 , 3.37999823, 3.39499816, 3.40999809,
3.42499803, 3.43999796, 3.45499789, 3.46999782, 3.48499775,
3.49999768])
[21]:
data_h2d.axes['mass'].centers # bin centers
[21]:
array([2.75750106, 2.772501 , 2.78750093, 2.80250086, 2.81750079,
2.83250072, 2.84750065, 2.86250059, 2.87750052, 2.89250045,
2.90750038, 2.92250031, 2.93750024, 2.95250018, 2.96750011,
2.98250004, 2.99749997, 3.0124999 , 3.02749983, 3.04249977,
3.0574997 , 3.07249963, 3.08749956, 3.10249949, 3.11749942,
3.13249936, 3.14749929, 3.16249922, 3.17749915, 3.19249908,
3.20749902, 3.22249895, 3.23749888, 3.25249881, 3.26749874,
3.28249867, 3.29749861, 3.31249854, 3.32749847, 3.3424984 ,
3.35749833, 3.37249826, 3.3874982 , 3.40249813, 3.41749806,
3.43249799, 3.44749792, 3.46249785, 3.47749779, 3.49249772])
[22]:
data_h2d.axes['mass'].widths # bin widths
[22]:
array([0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993,
0.01499993, 0.01499993, 0.01499993, 0.01499993, 0.01499993])
Multi dimensional
All this attributes are also already available in edges
, they are ready to be broadcasted. So they have the shape of (1, …, N, …, 1).
[23]:
data_h2d.axes.edges
data_h2d.axes['mass'].centers
data_h2d.axes['mass'].widths
areas = np.prod(data_h2d.axes.widths, axis=0)
print(f"areas = {areas}")
areas = [[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]
[0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536 0.00072536 0.00072536 0.00072536 0.00072536
0.00072536 0.00072536]]
Exercise: can you obtain the density?
use hist.values
or hist.views()
(the latter makes no copy, the former does).
[ ]:
Arithmetics
We can use the histograms to do math! We can multiply, add with each other or with scalars.
We can find the ratio between two histograms by dividing them
[24]:
data_df_bdt = data_df.query("BDT > 0.9")
data_bdt_h2d = hist.Hist(axis1, axis_bdt)
data_bdt_h2d.fill(data_df_bdt['Jpsi_M'], data_df_bdt['BDT']) # order based
[24]:
Regular(20, 0.026503, 0.993653, name='BDT')
Double() Σ=734.0 (735.0 with flow)
[25]:
ratio = data_bdt_h2d.project("mass") / data_h2d.project("mass")
[26]:
ratio.plot1d()
[26]:
[StairsArtists(stairs=<matplotlib.patches.StepPatch object at 0x7efc57ac82d0>, errorbar=None, legend_artist=None)]
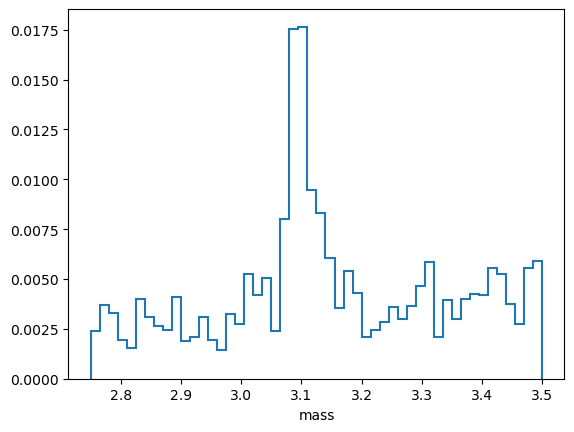
[27]:
ratio_large = ratio * 10
ratio_large.plot1d()
[27]:
[StairsArtists(stairs=<matplotlib.patches.StepPatch object at 0x7efc5496e850>, errorbar=None, legend_artist=None)]
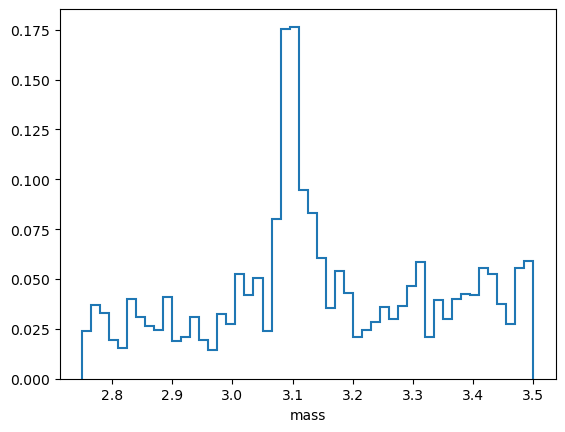
Exercise: use the subtraction to “remove” the signal from the data file using the BDT cut hist. This should be the same as using only “BDT<0.9”.
[ ]:
Weights
Weights are an essential part in HEP histograms and hist fully supports weigths. We can simply give an array of weights when filling the histogram.
We first need to specify the storage type to be of type Weight
in order to make sure we keep track of the weigths.
[28]:
weight = np.random.normal(1., 0.1, size=mc_df.shape[0])
storage = hist.storage.Weight()
mc_h2d = hist.Hist(axis1, axis_bdt, storage=storage).fill(BDT=mc_df['BDT'], mass=mc_df['Jpsi_M'], weight=weight) # using names
[29]:
mc_h2d
[29]:
Regular(20, 0.026503, 0.993653, name='BDT')
Weight() Σ=WeightedSum(value=119944, variance=121129) (WeightedSum(value=120004, variance=121190) with flow)
[30]:
mc_h2d.variances()
[30]:
array([[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 1.01219141e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 2.29985695e+00, 8.65509141e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
1.18022335e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 8.46378309e-01, 0.00000000e+00, 6.47978108e-01,
9.20287972e-01, 1.66505496e+00, 2.30821210e+00, 2.44905638e+01],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 1.30474650e+00, 3.22026031e+00, 0.00000000e+00,
6.81701892e+00, 3.08929143e+00, 7.86568598e+00, 3.80971830e+00,
5.10269454e+00, 1.34957078e+01, 7.80021568e+00, 1.24387450e+01,
1.57752594e+01, 2.30442911e+01, 4.04977516e+01, 1.02392478e+02],
[0.00000000e+00, 0.00000000e+00, 2.11252749e+00, 6.47032999e+00,
7.08283198e+00, 2.78664248e+01, 4.58121930e+01, 9.80247897e+01,
8.79469050e+01, 9.47459532e+01, 8.97525577e+01, 7.85411570e+01,
8.20304649e+01, 8.53514535e+01, 1.19828580e+02, 1.15933579e+02,
1.30510684e+02, 1.58610485e+02, 2.45068327e+02, 3.36688485e+02],
[0.00000000e+00, 0.00000000e+00, 1.52897610e+01, 7.87993093e+01,
1.70815542e+02, 3.18483462e+02, 5.98152042e+02, 9.56437890e+02,
9.65365400e+02, 8.71718729e+02, 8.12253764e+02, 7.68493441e+02,
7.31155667e+02, 6.85707211e+02, 6.94770814e+02, 7.13765823e+02,
6.86081848e+02, 7.39219353e+02, 9.85218760e+02, 9.84839415e+02],
[0.00000000e+00, 2.37547833e+00, 4.44922959e+01, 2.82072626e+02,
5.80864212e+02, 1.24330199e+03, 2.40154111e+03, 4.12924769e+03,
3.81122252e+03, 3.31588417e+03, 3.10537178e+03, 2.69236675e+03,
2.59943451e+03, 2.29319765e+03, 2.22818940e+03, 2.24146472e+03,
2.16911794e+03, 2.15300328e+03, 2.43780469e+03, 2.35498829e+03],
[0.00000000e+00, 8.36502895e-01, 6.34205349e+01, 2.76740083e+02,
6.99738879e+02, 1.39670757e+03, 2.81420876e+03, 4.72661931e+03,
4.39494418e+03, 3.96842680e+03, 3.68177926e+03, 3.18553553e+03,
2.97000975e+03, 2.59381988e+03, 2.78591978e+03, 2.41953396e+03,
2.46278776e+03, 2.49798789e+03, 2.72828053e+03, 2.63903062e+03],
[0.00000000e+00, 0.00000000e+00, 1.26514670e+01, 9.75226769e+01,
2.43513614e+02, 4.83535318e+02, 8.42561212e+02, 1.53077720e+03,
1.47950527e+03, 1.33652790e+03, 1.22833511e+03, 1.14915184e+03,
1.17257482e+03, 1.05404120e+03, 1.10150124e+03, 9.82553283e+02,
1.02777237e+03, 1.06488915e+03, 1.35315499e+03, 1.35151308e+03],
[0.00000000e+00, 0.00000000e+00, 9.00502194e-01, 6.66292209e+00,
2.36321569e+01, 4.02483729e+01, 8.93680745e+01, 1.33599807e+02,
1.78816237e+02, 1.54148138e+02, 1.64632702e+02, 1.35290399e+02,
1.59903912e+02, 1.51456514e+02, 2.08334100e+02, 1.83967702e+02,
1.94803922e+02, 2.63695077e+02, 3.51667381e+02, 4.49541745e+02],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 1.08657553e+00,
9.26712345e-01, 3.52022180e+00, 8.04106861e+00, 6.11264971e+00,
1.52563533e+01, 6.76462757e+00, 7.86933147e+00, 1.02128015e+01,
1.48114787e+01, 1.31810328e+01, 1.82502393e+01, 3.03983407e+01,
1.62721244e+01, 3.50096034e+01, 6.79896596e+01, 1.29531075e+02],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 1.21490401e+00, 0.00000000e+00,
1.09609973e+00, 0.00000000e+00, 0.00000000e+00, 2.74776087e+00,
1.67217321e+00, 3.95254457e+00, 1.26225347e+01, 2.56102488e+01],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 2.06630935e+00, 1.15670169e+01],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 2.62635336e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 2.88504803e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 1.20073802e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00],
[0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00]])
Exercise: implement a function that calculates a weighted chi2 using two histograms.
[ ]: